Ignoring or avoiding the accept-encoding: gzip, deflate, br
November 8, 2024 | by Cícero Fabio
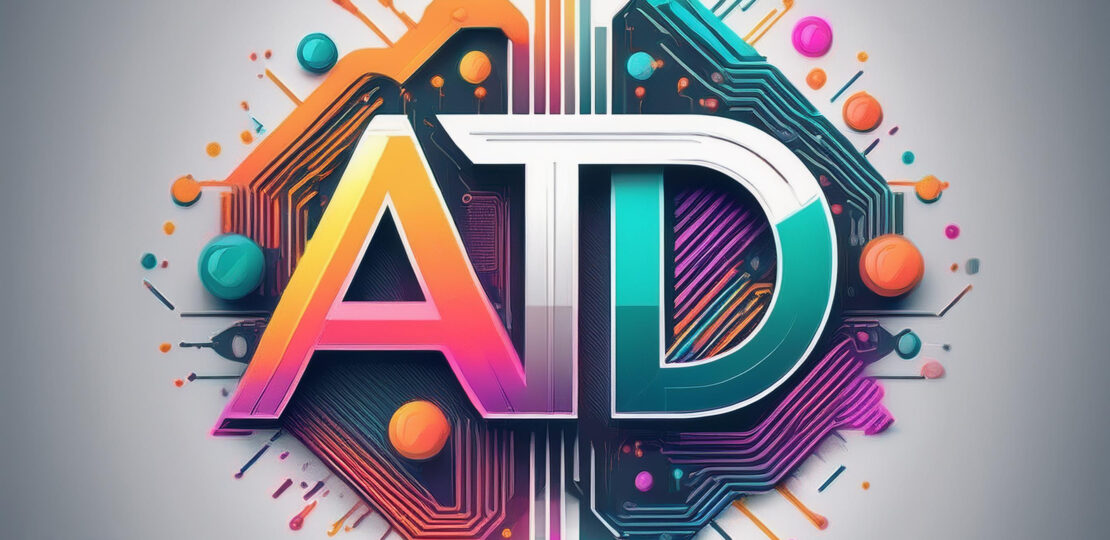
In a Spring Boot application, if you want to ignore or avoid the Accept-Encoding
header (which commonly includes values like gzip
, deflate
, or br
), you can configure your application to respond without applying these encodings. Here are several approaches you can take:
1. Set Response Headers
You can modify the response headers to ensure that the Content-Encoding
is not applied. This can be done in a @ControllerAdvice
or directly in your controller methods.
import org.springframework.http.HttpHeaders;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
@ControllerAdvice
@RestController
public class CustomHeaderAdvice {
@ResponseBody
public ResponseEntity<String> handleResponse(String body) {
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_ENCODING, "identity"); // No encoding
return ResponseEntity.ok().headers(headers).body(body);
}
}
2. Disable Compression Globally
You can disable response compression in your Spring Boot application by modifying the application properties. Add the following line to your application.properties
or application.yml
:
# application.properties
spring.http.gzip.enabled=false
or
# application.yml
spring:
http:
gzip:
enabled: false
3. Use a Filter
If you need more control, you can implement a Filter
to modify the response headers:
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class NoGzipFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletResponse httpResponse = (HttpServletResponse) response;
httpResponse.setHeader("Content-Encoding", "identity"); // No encoding
chain.doFilter(request, response);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// Initialization code if needed
}
@Override
public void destroy() {
// Cleanup code if needed
}
}
Then, register the filter in your Spring Boot application:
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class FilterConfig {
@Bean
public FilterRegistrationBean<NoGzipFilter> noGzipFilter() {
FilterRegistrationBean<NoGzipFilter> registrationBean = new FilterRegistrationBean<>();
registrationBean.setFilter(new NoGzipFilter());
registrationBean.addUrlPatterns("/*"); // Apply to all URLs
return registrationBean;
}
}
Additional Notes
- Client-Side: Ensure that the clients making requests to your application are also configured not to request compression if they can control that aspect.
- Testing: After implementing these changes, you should test your application to confirm that the
Accept-Encoding
header is being handled as you expect.
By following one of these methods, you can effectively ignore or handle the Accept-Encoding
header in your Spring Boot application.