Implementing User Role Management with React and MongoDB
September 19, 2024 | by Cícero Fabio
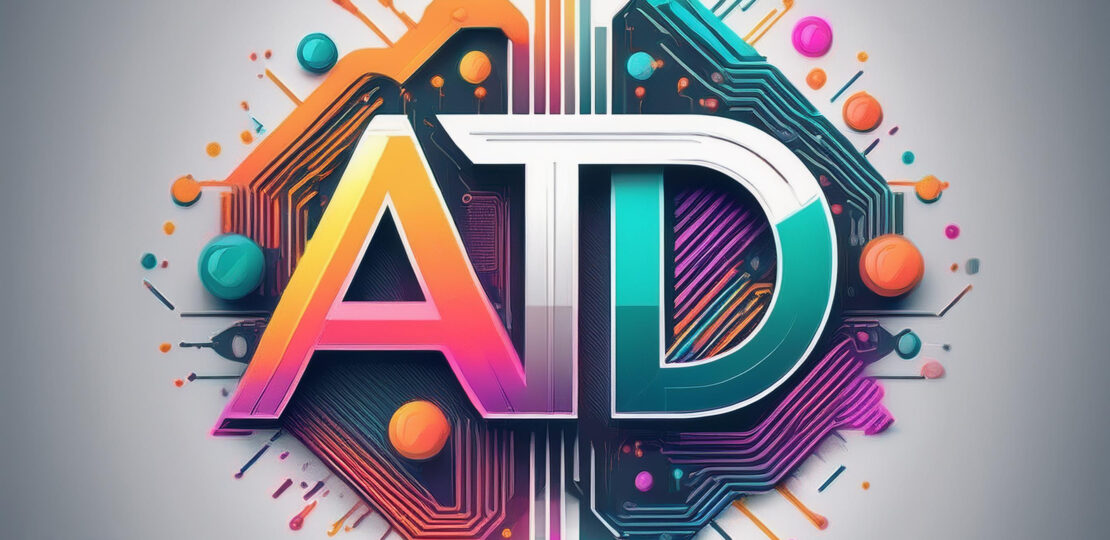
Simple React project structure that allows controlling access roles for a User
document in MongoDB. We’ll create a small application that demonstrates how to manage users and their associated insurance policies.
Project Setup
- Create React App:
npx create-react-app insurance-policy-manager
cd insurance-policy-manager
- Install Axios (for API requests):
npm install axios
- Set Up MongoDB:
You can use MongoDB Atlas or a local MongoDB instance. Ensure you have a collection calledusers
with documents structured as shown in your example.
Backend Setup (Node.js + Express)
Create a simple backend for managing users.
- Create a new folder for the backend:
mkdir backend
cd backend
npm init -y
npm install express mongoose cors body-parser
- Create a simple server:
Create a file namedserver.js
in thebackend
folder.
// backend/server.js
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const bodyParser = require('body-parser');
const app = express();
app.use(cors());
app.use(bodyParser.json());
mongoose.connect('mongodb://localhost:27017/insurance', { useNewUrlParser: true, useUnifiedTopology: true });
const userSchema = new mongoose.Schema({
name: String,
cnpj: String,
policies: [String]
});
const User = mongoose.model('User', userSchema);
// Get user by CNPJ
app.get('/api/users/:cnpj', async (req, res) => {
const user = await User.findOne({ cnpj: req.params.cnpj });
res.json(user);
});
// Update user policies
app.put('/api/users/:cnpj', async (req, res) => {
const { policies } = req.body;
const user = await User.findOneAndUpdate({ cnpj: req.params.cnpj }, { policies }, { new: true });
res.json(user);
});
app.listen(5000, () => {
console.log('Server is running on http://localhost:5000');
});
- Run the backend:
node server.js
Frontend Setup (React)
- Create Components:
Create a folder namedcomponents
inside thesrc
directory in your React app. - UserPolicyManager.js:
Create a file namedUserPolicyManager.js
in thecomponents
folder.
// src/components/UserPolicyManager.js
import React, { useState } from 'react';
import axios from 'axios';
const UserPolicyManager = () => {
const [cnpj, setCnpj] = useState('');
const [user, setUser] = useState(null);
const [newPolicies, setNewPolicies] = useState([]);
const fetchUser = async () => {
const response = await axios.get(`http://localhost:5000/api/users/${cnpj}`);
setUser(response.data);
};
const updatePolicies = async () => {
await axios.put(`http://localhost:5000/api/users/${cnpj}`, { policies: newPolicies });
fetchUser(); // Refresh the user data
};
return (
<div>
<h1>User Policy Manager</h1>
<input
type="text"
placeholder="Enter CNPJ"
value={cnpj}
onChange={(e) => setCnpj(e.target.value)}
/>
<button onClick={fetchUser}>Fetch User</button>
{user && (
<div>
<h2>User: {user.name}</h2>
<p>CNPJ: {user.cnpj}</p>
<p>Policies: {user.policies.join(', ')}</p>
<input
type="text"
placeholder="New Policies (comma-separated)"
onChange={(e) => setNewPolicies(e.target.value.split(',').map(s => s.trim()))}
/>
<button onClick={updatePolicies}>Update Policies</button>
</div>
)}
</div>
);
};
export default UserPolicyManager;
- Update App.js:
Modifysrc/App.js
to include theUserPolicyManager
component.
// src/App.js
import React from 'react';
import UserPolicyManager from './components/UserPolicyManager';
function App() {
return (
<div className="App">
<UserPolicyManager />
</div>
);
}
export default App;
Run the Application
- Start React App:
npm start
Final Note
- Ensure your MongoDB is running and accessible.
- This example provides a simple interface to fetch and update user policies based on CNPJ.
- You can further enhance the app by adding error handling, validation, and styling as needed.
Conclusion
With this setup, you have a basic application to control access roles for users and their insurance policies. You can extend it further based on your requirements!